Flicker Image Search API Demo
![]() |
Flicker API |
<html>
<head>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<title>Flicker Image Search API Demo</title>
</head>
<body>
Search for <b>girls, dogs, cakes</b> ...etc)
<br />
<input id="searchterm" />
<button id="search">search</button>
<div id="results"></div>
<script>
$("#search").click(function(){
$.getJSON("http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?",
{
tags: $("#searchterm").val(),
format: "json"
},
function(data) {
$.each(data.items, function(i,item){
$("<img/>").attr("src", item.media.m).prependTo("#results");
if ( i == 10 ) return false;
});
});
});
</script>
</body>
</html>
How to access Session Bean from JSP in GlassFish
First add a reference in deployment descriptor to the session bean.
<ejb-local-ref>
<ejb-ref-name>AccountTypeFacadeRef</ejb-ref-name>
<ejb-ref-type>Session</ejb-ref-type>
<local>com.my.ejb.AccountTypeFacade</local>
<ejb-link>BankApp-ejb.jar#AccountTypeFacade</ejb-link>
</ejb-local-ref>
is optional.
In JSP use JNDI lookup to find the resource.
<%
String prefix = "java:comp/env/";
String ejbRefName = "AccountTypeFacadeRef";
String jndiUrl = prefix + ejbRefName;
javax.naming.Context ctx = new javax.naming.InitialContext();
AccountTypeFacade atf = ( AccountTypeFacade ) ctx.lookup( jndiUrl );
List<AccountType> accountTypeList = atf.findAll();
%>
Swing File Chooser Demo
package main;
import java.awt.BorderLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JDialog;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class SwingFileChooser extends JPanel implements ActionListener{
private static final String newLine = "\n";
private JButton openButton,saveButton;
private JTextArea log;
private JFileChooser fc;
public SwingFileChooser(){
super(new BorderLayout());
initComponents();
}
private void initComponents(){
log = new JTextArea();
log.setMargin(new Insets(5, 5, 5, 5));
log.setEditable(false);
JScrollPane jsp = new JScrollPane(log);
fc = new JFileChooser();
fc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES);
openButton = new JButton("Open File");
openButton.addActionListener(this);
saveButton = new JButton("Save File");
saveButton.addActionListener(this);
JPanel buttonPanel = new JPanel();
buttonPanel.add(openButton);
buttonPanel.add(saveButton);
add(buttonPanel,BorderLayout.NORTH);
add(jsp,BorderLayout.CENTER);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource() == openButton){
int returnVal = fc.showOpenDialog(this);
if(returnVal == JFileChooser.APPROVE_OPTION){
File file = fc.getSelectedFile();
log.append("Opening " + file.getName() + newLine);
}else{
log.append("cancelled file opening" + newLine);
}
log.setCaretPosition(log.getDocument().getLength());
}else if(e.getSource() == saveButton){
int returnVal = fc.showSaveDialog(this);
if(returnVal == JFileChooser.APPROVE_OPTION){
File file = fc.getSelectedFile();
log.append("Saving " + file.getName() + newLine);
}else{
log.append("cancelled file saving" + newLine);
}
log.setCaretPosition(log.getDocument().getLength());
}
}
private static void createGUI(){
JFrame.setDefaultLookAndFeelDecorated(true);
JDialog.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("SwingFileChooser");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JComponent newContentPane = new SwingFileChooser();
newContentPane.setOpaque(true);
frame.setContentPane(newContentPane);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
createGUI();
}
});
}
}
OpenSource Directions API Demo - YOURS Navigation API
In this tutorial, I 'll introduce you to YOURS, an OpenSource directions API. There are few OpenSource directions APIs available such as MapQuest, GeoSmart, Nominatim in addition to YOURS. However in my opinion, YOURS is the best and a good alternative to Google Directions which is the most popular Directions provider obviously.
The flexibility of usage has given more power in YOURS over other APIs in the same family. MapQuest is nice but it is not working for many geographical locations of the world other than North America and Europe. Nominatim is poor in its functional level and has less support for geographical diversity.
According to YOURS doucmentation, the API provide following features.
- Generate fastest or shortest routes in different modes:
- using all available roads for Car, Bicycle and Pedestrians .
- using only national, regional or local cycle routes/networks for Bicycle.
- Unlimited via points (waypoints) to make complex routes.
- Drag and drop waypoints moving.
- Drag and drop waypoint ordering.
- Geolocation: Lookup street- and placenames to determine their coordinates.
- Reverse geolocation: Lookup coordinates to determing their street- and placenames.You can read other features in doucmentation.
Example URL
http://www.yournavigation.org/api/1.0/gosmore.php?format=kml&flat=52.215676&flon=5.963946&tlat=52.2573&tlon=6.1799&v=motorcar&fast=1&layer=mapnik
This tutorial provides sample code for accessing the API in JavaScript. As JavaScript does not allow cross domain requests we 're using a proxy which accepts requests from client, forward it to the API and send back server response. The proxy is written in PHP using CURL which is more safe than file_get_contents() and similar content loading functions.
We are using OpenLayers map with a vector layer which is going get features from server response. The route is drawn on map using vector data.
The API also provides travelling time, distance and turn by turn directions.
Demo
client.html
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<meta name="description" content="OpenSource Directions API Demo - YOURS Navigation API">
<title>OpenSource Directions API Demo</title>
<link rel="stylesheet" href="http://demos.site11.com/assets/css/style.css">
<script src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script src="http://openlayers.org/api/OpenLayers.js"></script>
var lon = 0;
var lat = 0;
var zoom = 0;
var wgs84 = new OpenLayers.Projection("EPSG:4326");
var mercator = new OpenLayers.Projection("EPSG:900913");
$(document).ready(function(){
layer = new OpenLayers.Layer.OSM("OSM");
geojson_layer = new OpenLayers.Layer.Vector("GeoJSON", {
styleMap: new OpenLayers.StyleMap({
strokeColor: "#F00",
projection: mercator
}),
strategies: [new OpenLayers.Strategy.Fixed()],
protocol: new OpenLayers.Protocol.HTTP({
url: 'proxy.php?flat=6.9344&flon=79.8428&tlat=7.2844590&tlon=80.637459&v=motorcar&fast=1&layer=mapnik',
format: new OpenLayers.Format.GeoJSON()
})
});
var options = {
div : "map",
projection : wgs84,
units: "dd",
numZoomLevels : 7
};
var map = new OpenLayers.Map(options);
map.addLayers([layer,geojson_layer]);
map.setCenter(new OpenLayers.LonLat(79.8428, 6.9344).transform(wgs84,mercator), 12);
//Get Directions
$.ajax({
type: 'GET',
dataType: 'json',
url: 'proxy.php?flat=6.9344&flon=79.8428&tlat=7.2844590&tlon=80.637459&v=motorcar&fast=1&layer=mapnik',
cache: false,
success: function(response){
$("#travel_time").html(response.properties.traveltime);
$("#distance").html(response.properties.distance + ' miles');
$("#directions").html(response.properties.description);
},error: function(){
}
});
});
</script>
<style type="text/css">
#map{
width: 800px;
height: 400px;
border: 2px solid black;
padding:0;
margin:0;
}
</style>
</head>
<body>
<div id="map" style="width: 700px; height: 300px;margin-bottom : 50px;" align="center"></div>
<p><b>Travel Time</b></p><div id="travel_time"></div><br>
<p><b>Distance</b></p><div id="distance"></div><br>
<p><b>Directions</b></p><div id="directions"></div>
</body>
</html>
Proxy.php
<?php
$flat = $_GET['flat'];
$flon = $_GET['flon'];
$tlat = $_GET['tlat'];
$tlon = $_GET['tlon'];
$v = $_GET['v'];
$fast = $_GET['fast'];
$layer = $_GET['layer'];
$myURL = "http://www.yournavigation.org/api/1.0/gosmore.php?format=geojson&instructions=1&flat=".$flat."&flon=".$flon."&tlat=".$tlat."&tlon=".$tlon."&v=".$v."&fast=".$fast."&layer=".$layer;
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_RETURNTRANSFER => 1,
CURLOPT_URL => $myURL
));
$resp = curl_exec($curl);
curl_close($curl);
echo $resp;
?>
How to store an array in localStorage
![]() |
HTML5 LocalStorage |
This tutorial assumes that you are already familiar with HTML5 localStorage.
Although HTML5 localStorage is very useful, its usage is restricted to key value mechanism. Key and value is stored in string format despite what we need. For an instance, though you can specify a boolean type (either true or false) as the value, it is stored as a string.
What if we need to store multiple items as the value for a certain key. This can be little bit tricky if you gonna place an array directly as the value. When we scratch the surface of localStorage basic principles this is not possible. However you can come up with your own solutions that might work for you.
This tutorial demonstrates a simple way of handling above problem using json. We gonna store array as a json string. So nothing new here.
First add jQuery library as we are using some jQuery functions.
<script type="text/javascript">
var favorites_str = localStorage.getItem('my_favorites');
if(favorites_str == null) {
favorites = [];
favorites.push({ "name":"keshi", "id":"6" });
} else{
favorites = JSON.parse(favorites_str);
favorites.push({ "name":"sara", "id":"6" });
}
localStorage.setItem('my_favorites',JSON.stringify(favorites));
</script>
To verify that above script is functioning please copy and run below script. It will make things easy, if you put this code in a separate file.
<script type="text/javascript">
var data = localStorage.getItem('my_favorites');
if(data == null){
alert("0 favorites");
}else{
favorites = JSON.parse(data);
$.each(favorites, function(index,item){
alert(item.name);
});
}
</script>
OpenSource PHP Graph libraries
![]() |
PHP Graphs |
There are so many PHP graph projects which you can find on internet. At the time of writing this post, JpGraph, phpgraphlib and pChart should appear in top Google search results. In my experience, phpgraphlib is developer-friendly and easy to use whereas JpGraph is more robust than others. pChart seems to be little bit outdated though they have updated their project.
JpGraph
phpgraphlib
Create Charts with jFreeChart
This tutorial is all about creating graphs. There are several tools for generating graphs without any hassle. Programming a graph from scratch might little be tricky as it also deals with some graphics. Using a tool or library reduces the development time a lot. So we gonna use an OpenSource chart tool named jFreeChart for Java development.
Creating a graph with jFreeChart is just a matter of entering the required graph data and a little piece of code. Therefore, as the CodeZone4 norm I just go ahead with a 3D bar graph implementation using jFreeChart.
You can find source code for other types of graph on the internet.
First download required JARs from jFreeChart and add them in your project's classpath.
import org.jfree.chart.*;
import org.jfree.data.category.*;
import org.jfree.data.general.DefaultPieDataset;
import org.jfree.data.xy.*;
import org.jfree.data.*;
import org.jfree.chart.renderer.category.*;
import org.jfree.chart.plot.*;
import java.awt.*;
public class Main {
public static void main(String[] args) {
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.setValue(3780, "A", "2008");
dataset.setValue(5000, "B", "2008");
dataset.setValue(6500, "A", "2009");
dataset.setValue(6000, "B", "2009");
dataset.setValue(9000, "A", "2010");
dataset.setValue(10000, "B", "2010");
JFreeChart chart = ChartFactory.createBarChart3D("Annual Income Analysis", "Year", "Income",
dataset, PlotOrientation.VERTICAL, true, true, false);
chart.setBackgroundPaint(Color.yellow);
chart.getTitle().setPaint(Color.blue);
CategoryPlot p = chart.getCategoryPlot();
p.setRangeGridlinePaint(Color.red);
ChartFrame frame1 = new ChartFrame("Income Data", chart);
frame1.setVisible(true);
frame1.setSize(300, 300);
}
}
Develop Voice Applications with VoiceXML
![]() |
voice xml |
Web pages consist of HTML that is rendered into a visible page by a Web browser. Similarly, a voice application consists of XML (VoiceXML, CCXML, or CallXML) which becomes an interactive voice application when processed by the Voxeo Corporation VoiceCenter network. All you need to do is write the application's XML, map it to a phone number in the Voxeo Application Manager, and give it a call.
https://evolution.voxeo.com/
Sample vxml file
<?xml version="1.0" encoding="UTF-8"?>
<vxml version="2.0" xmlns="http://www.w3.org/2001/vxml">
<form id="login">
<field name="phone_number" type="phone">
<prompt>Please say your complete phone number</prompt>
</field>
<field name="pin_code" type="digits">
<prompt>Please say your PIN code</prompt>
</field>
<block>
<submit next="http://www.example.com/servlet/login"
namelist="phone_number pin_code"/>
</block>
</form>
</vxml>
Getting started with VoiceXML 2.0
Simple CRUD with MongoDB and PHP
![]() |
PHP CRUD with MongoDB |
Today we gonna make our hands dirty with some MongoDB stuff. MongoDB is a no-SQL type, schema-less database. Such databases can be very useful in situations where there is much more user-generated content. You should understand where you use MongoDB or Apache CouchDB rather than traditional relational databases. You may want to google for getting more details about MongoDB if you gonna try this tutorial as a newbie.
First download and install MongoDB in your environment.
As we are trying this tutorial in PHP, you should download mongo driver for PHP also.
Edit php.ini so that it can load mongo driver. Add this line(in Windows)
extension=php_mongo.dll
Now we are good to go.
Run MongoDB server and start Mongo shell.
First you need to create a database and add a database user. If this is the first time you 're going to create a database user, first you need to create an admin user. Read documentation for more details.
This tutorial is all about a simple CRUD application using MongoDB. In this application a blog post scenario is used for demonstrating CRUD operations. This scenario is very popular in MongoDB Hello World tutorials.
This tutorial does not include complete source code.
DB class
class DB {
const DBHOST = 'localhost';
const DBUSER = 'codezone4';
const DBPWD = '123';
const DBPORT = 27017;
const DBNAME = 'blog_db';
private static $instance;
public $connection;
public $databse;
private function __construct() {
$connection_string = sprintf('mongodb://%s:%s@%s:%d/%s', DB::DBUSER, DB::DBPWD, DB::DBHOST, DB::DBPORT, DB::DBNAME);
try {
$this->connection = new Mongo($connection_string);
$this->databse = $this->connection->selectDB(DB::DBNAME);
} catch (MongoConnectionException $e) {
throw $e;
}
}
static public function instantiate(){
if(!isset(self::$instance)){
$class = __CLASS__;
self::$instance = new $class;
}
return self::$instance;
}
public function get_collection($name){
return $this->databse->selectCollection($name);
}
}
Add New Post
include 'db.class.php';
if(isset($_POST['add_post'])){
$title = $_POST['title'];
$content = $_POST['content'];
if(!empty($title) && !empty($content)){
$mongo = DB::instantiate();
$post_collection = $mongo->get_collection('posts');
$post = array(
'_id' => hash('sha1', time() . $title),
'title' => $title,
'content' => $content,
'created_on' => new MongoDate()
);
$post_id = $post_collection->insert($post,array('safe'=>TRUE));
header('Location:../dashboard.php');
}
}
jQueryMobile with Google Map
![]() |
jQueryMobile with Google Map |
This demo is based on jQuery UI plugin for Google Maps.
<!doctype html>
<html lang="en">
<head>
<title>jQuery mobile with Google maps</title>
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.2.0/jquery.mobile-1.2.0.min.css" />
<script src="http://code.jquery.com/jquery-1.8.2.min.js"></script>
<script src="http://code.jquery.com/mobile/1.2.0/jquery.mobile-1.2.0.min.js"></script>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?v=3&sensor=false&language=en"> </script>
<script type="text/javascript" src="http://jquery-ui-map.googlecode.com/svn/trunk/ui/min/jquery.ui.map.min.js"></script>
<script type="text/javascript">
var colombo = new google.maps.LatLng(26.5727,73.8390);
var delhi = new google.maps.LatLng(28.6100,77.2300);
mobileDemo = { 'center': '28.6100,77.2300', 'zoom': 12 };
function initialize() {
$('#map_canvas').gmap({ 'center': mobileDemo.center, 'zoom': mobileDemo.zoom, 'disableDefaultUI':false });
$('#map_canvas').gmap('addMarker', { 'position': delhi } );
}
$(document).on("pageinit", "#basic-map", function() {
initialize();
});
$(document).on('click', '.add-markers', function(e) {
e.preventDefault();
$('#map_canvas').gmap('addMarker', { 'position': colombo } );
});
</script>
</head>
<body>
<div id="basic-map" data-role="page">
<div data-role="header">
<h1><a data-ajax="false" href="#">jQuery mobile with Google</a></h1>
<a data-rel="back">Back</a>
</div>
<div data-role="content">
<div style="padding:1em;">
<div id="map_canvas" style="height:350px;"></div>
</div>
<a href="#" data-role="button" data-theme="b">Add Some More Markers</a>
</div>
</div>
</body>
</html>
Find Visitor's IP address in PHP
Relying on $_SERVER['REMOTE_ADDR'] to find your client's ip address is not always good. Looking for a wide solution?
Then use this function.
Then use this function.
function get_ip() {
$ip = '';
if ($_SERVER['HTTP_CLIENT_IP'])
$ip = $_SERVER['HTTP_CLIENT_IP'];
else if($_SERVER['HTTP_X_FORWARDED_FOR'])
$ip = $_SERVER['HTTP_X_FORWARDED_FOR'];
else if($_SERVER['HTTP_X_FORWARDED'])
$ip = $_SERVER['HTTP_X_FORWARDED'];
else if($_SERVER['HTTP_FORWARDED_FOR'])
$ip = $_SERVER['HTTP_FORWARDED_FOR'];
else if($_SERVER['HTTP_FORWARDED'])
$ip = $_SERVER['HTTP_FORWARDED'];
else if($_SERVER['REMOTE_ADDR'])
$ip = $_SERVER['REMOTE_ADDR'];
else
$ip = '';
return $ip;
}
Sending Emails with Swift Mailer
![]() |
Swift Mailer |
"Swift Mailer integrates into any web app written in PHP 5, offering a flexible and elegant object-oriented approach to sending emails with a multitude of features."
Download Documentation
<!--?php require_once 'swiftmailer/lib/swift_required.php'; // Create the Transport //SMTP /*$transport = Swift_SmtpTransport::newInstance('smtp.example.org', 25) --->setUsername('your username')
->setPassword('your password')
;
*/
// Sendmail
//$transport = Swift_SendmailTransport::newInstance('/usr/sbin/sendmail -bs');
// Mail
$transport = Swift_MailTransport::newInstance();
// Create the Mailer using your created Transport
$mailer = Swift_Mailer::newInstance($transport);
// Create a message
$message = Swift_Message::newInstance('Wonderful Subject')
->setFrom(array('john@doe.com' => 'John Doe'))
->setTo(array('receiver@domain.org', 'other@domain.org' => 'A name'))
->setBody('Here is the message itself')
;
// Send the message
$result = $mailer->send($message);
?>
jQuery UI Dialog with Custom Buttons
![]() |
jQuery UI Dialog |
<div id="dialog" title="Delete">
<p>Are you sure that you want to delete this division?</p>
</div>
$("#dialog").dialog({
modal: true,
resizable: false,
width: 800,
buttons: [{
text: "Yes",
click: function() {
alert("Clicked Yes");
}
},
{
text: "No",
click: function() {
$(this).dialog("close");
}
}]
});
HTML5 SQL Database Tutorial
![]() |
HTML5 Data Storage |
HTML5 supports client side data storage. There are several types of storing data with HTML5
1. local storage through localStorage object
2. session storage through sessionStorage object
3. SQL based database
This tutorial demonstrates how to manage a client side SQL based database with HTML5.
Demo
<!DOCTYPE html>
<html>
<head>
<meta name=viewport content="user-scalable=no,width=device-width" />
<link rel="stylesheet" href="css/jquery.mobile-1.1.0.min.css" />
<script src="js/jquery-1.7.2.min.js"></script>
<script src="js/jquery.mobile-1.1.0.min.js"></script>
</head>
<body>
<div data-role=page id=home>
<div data-role=header>
<h1>Home</h1>
</div>
<div data-role="content">
<a href="#" data-role="button" id="create"> Create table </a>
<a href="#" data-role="button" id="remove"> Delete table </a>
<span> Item </span>
<input type="text" id="item">
<span> Quantity </span>
<input type="text" id="quantity">
<a href="#" data-role="button" id="insert">Insert item</a>
<a href="#" data-role="button" id="list">List items </a>
<ul data-inset="true" data-role="listview" id="itemlist"></ul>
</div>
</div>
<script>
var db = openDatabase ("itemDB", "1.0", "itemDB", 65535);
$("#create").bind ("click", function (e)
{
db.transaction (function (transaction)
{
var sql = "CREATE TABLE items " +
" (id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT, " +
"item VARCHAR(100) NOT NULL, " +
"quantity int(2) NOT NULL)"
transaction.executeSql (sql, undefined, function ()
{
alert ("Table created");
},error
);
});
});
$("#remove").bind ("click", function (e)
{
if (!confirm ("Delete table?", "")) return;;
db.transaction (function (transaction)
{
var sql = "DROP TABLE items";
transaction.executeSql (sql, undefined, success, error);
});
});
$("#insert").bind ("click", function (event)
{
var item = $("#item").val ();
var quantity = $("#quantity").val ();
db.transaction (function (transaction)
{
var sql = "INSERT INTO items (item, quantity) VALUES (?, ?)";
transaction.executeSql (sql, [item, quantity], function ()
{
alert ("Item created!");
}, error);
});
});
$("#list").bind ("click", function (event)
{
$("#itemlist").children().remove()
db.transaction (function (transaction)
{
var sql = "SELECT * FROM items";
transaction.executeSql (sql, undefined,
function (transaction, result)
{
if (result.rows.length)
{
for (var i = 0; i < result.rows.length; i++)
{
var row = result.rows.item (i);
var item = row.item;
var quantity = row.quantity;
$("#itemlist").append("<li>" + item + " - " + quantity + "</li>");
}
}
else
{
$("#itemlist").append("<li> No items </li>");
}
}, error);
});
});
function success ()
{
}
function error (transaction, err)
{
alert ("DB error : " + err.message);
return false;
}
</script>
</body>
</html>
Please note that Firefox does not support this type of web SQL databases. If you try this in a FF browser, it will throw "ReferenceError: openDatabase is not defined".
OpenLayers GeoJSON Vector Layer
![]() |
Geojson |
Let's see how we can add a vector layer to OpenLayers map with the help of GeoJSON response received from the server. Web Server can produce response in GML, GeoJSON, XML...etc after running a spatial query.
OpenLayers has Format classes for interpreting each kind of response.
This response consists of raw data which should be converted to features and added to a vector layer in order to render with OpenLayers.
var newLayer = new OpenLayers.Layer.Vector("Filtered by Zoom Level", {
strategies: [new OpenLayers.Strategy.BBOX(),
],
protocol: new OpenLayers.Protocol.HTTP({
url: "http://localhost/test/a.php?start=79.891119%207.078768&end=81.000609%207.940130&method=SPD",
format: new OpenLayers.Format.GeoJSON({
externalProjection: new OpenLayers.Projection("EPSG:4326"),
internalProject: new OpenLayers.Projection("EPSG:900913")
})
}),
//filter: filter,
// styleMap: styleMap,
format: new OpenLayers.Format.GeoJSON({
externalProjection: new OpenLayers.Projection("EPSG:4326"),
internalProject: new OpenLayers.Projection("EPSG:900913")
})
});
map.addLayers([newLayer]);
Another Way
var newLayer = new OpenLayers.Layer.Vector("SPD", {
isBaseLayer: false,
styleMap: new OpenLayers.StyleMap({'default':{
strokeColor: "#F00",
strokeOpacity: 1,
strokeWidth: 2,
fillColor: "#FF5500",
fillOpacity: 0.5,
label : "${ad}",
fontSize: "8px",
fontFamily: "Courier New, monospace",
labelXOffset: "0.5",
labelYOffset: "0.5"
}})
});
OpenLayers.Request.GET({
url: "http://localhost/test/a.php?start=79.891119%207.078768&end=81.000609%207.940130&method=SPD",
headers: {'Accept':'application/json'},
success: function (req)
{
var g = new OpenLayers.Format.GeoJSON();
var feature_collection = g.read(req.responseText);
newLayer.destroyFeatures();
newLayer.addFeatures(feature_collection);
}
});
map.addLayers([newLayer]);
How to split ul into multiple columns
HTML Email with Codeigniter
Codeigniter configuration for PostgreSQL Database
![]() |
Codeigniter configuration for PostgreSQL Database |
First enable Postgresql extension in php.ini
extension=php_pgsql.dll
You also can enable Postgresql extension for PDO as well.
extension=php_pdo_pgsql.dll
If you forgot to enable this you may come across following error.
A PHP Error was encountered Severity: Warning Message: require_once(C:/www/system/database/drivers/postgres/postgres_driver.php) [function.require-once]: failed to open stream: No such file or directory Filename: database/DB.php Line Number: 138
Now opendatabase configuration file. You should enter correct settings for connecting with your PostgreSQL database here.
Sample config file.
$db['default']['hostname'] = 'localhost';
$db['default']['username'] = 'postgres';
$db['default']['password'] = 'postgres';
$db['default']['database'] = 'abc_gis';
$db['default']['dbdriver'] = 'postgre';
$db['default']['port'] = 5432;
$db['default']['dbprefix'] = '';
$db['default']['pconnect'] = TRUE;
$db['default']['db_debug'] = TRUE;
$db['default']['cache_on'] = FALSE;
$db['default']['cachedir'] = '';
$db['default']['char_set'] = 'utf8';
$db['default']['dbcollat'] = 'utf8_general_ci';
$db['default']['swap_pre'] = '';
$db['default']['autoinit'] = TRUE;
$db['default']['stricton'] = FALSE;
Codeigniter Facebook Login Tutorial using Facebook PHP SDK
![]() |
Codeigniter Facebook Login Tutorial using Facebook PHP SDK |
Welcome to my new Codeigniter tutorial for Facebook Login. I assume that you are familar with Codeigniter framework before starting the tutorial. However, you can adopt the source code to use in native PHP application if you are not interested in CI. There is another alternative. Previously, I have published two posts related with Facebook Login. You can also refer those tutorials.
Facebook OAUTH dialog with new Graph API
AJAX Facebook Connect Demo
First you need to create a Facebook application.
Visit this link to create new app.
This is a straight-forward process.
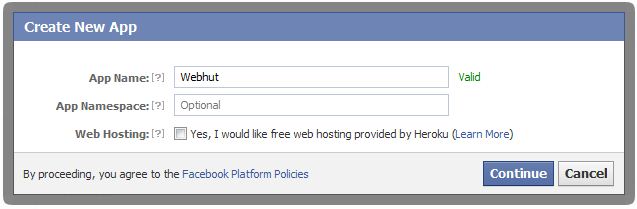
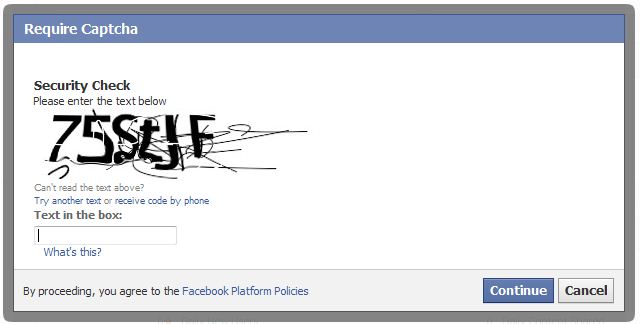
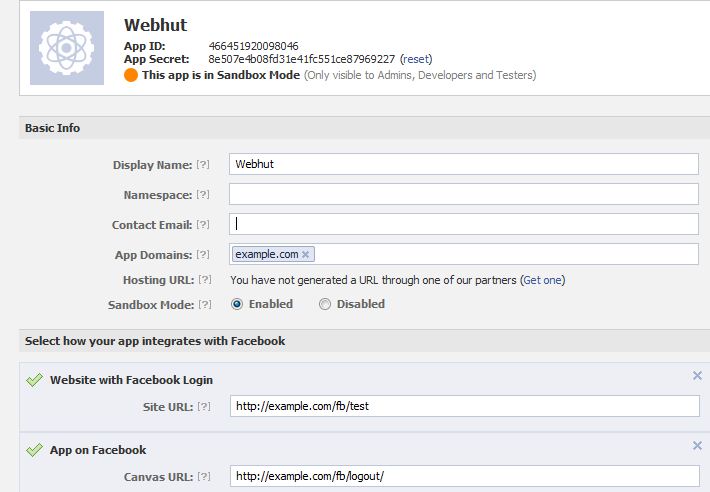
You need to get the App ID and App Secret of your application.
First create a config file to store App ID and App Secret.
config_facebook.php
$config['appID'] = 'YOUR_APP_ID';
$config['appSecret'] = 'YOUR_APP_SECRET';
Add a controller that handles Facebook login and logout.
fb.php
<?php
if (!defined('BASEPATH'))
exit('No direct script access allowed');
//include the facebook.php from libraries directory
require_once APPPATH . 'libraries/facebook/facebook.php';
class Fb extends CI_Controller {
public function __construct() {
parent::__construct();
$this->config->load('config_facebook');
}
public function index() {
$this->load->view('head');
$this->load->view('fb');
$this->load->view('footer');
}
public function logout() {
$signed_request_cookie = 'fbsr_' . $this->config->item('appID');
setcookie($signed_request_cookie, '', time() - 3600, "/");
$this->session->sess_destroy(); //session destroy
redirect('/fb/index', 'refresh'); //redirect to the home page
}
public function fblogin() {
$facebook = new Facebook(array(
'appId' => $this->config->item('appID'),
'secret' => $this->config->item('appSecret'),
));
// We may or may not have this data based on whether the user is logged in.
// If we have a $user id here, it means we know the user is logged into
// Facebook, but we don't know if the access token is valid. An access
// token is invalid if the user logged out of Facebook.
$user = $facebook->getUser(); // Get the facebook user id
$profile = NULL;
$logout = NULL;
if ($user) {
try {
$profile = $facebook->api('/me'); //Get the facebook user profile data
$access_token = $facebook->getAccessToken();
$params = array('next' => base_url('fb/logout/'), 'access_token' => $access_token);
$logout = $facebook->getLogoutUrl($params);
} catch (FacebookApiException $e) {
error_log($e);
$user = NULL;
}
$data['user_id'] = $user;
$data['name'] = $profile['name'];
$data['logout'] = $logout;
$this->session->set_userdata($data);
redirect('/fb/test');
}
}
public function test() {
$this->load->view('test');
}
}
/* End of file fb.php */
/* Location: ./application/controllers/fb.php */
In this tutorial, I'm using Facebook JavaScript SDK to load the oauth dialog. You need to add the App ID in following code to initiate the SDK successfully.
Reverse Geocoding with Google Map API
Demo
If you know the latitude and longitude of a particular location, you can get the relevant address details such as city, state, region, country...etc. This process is known as Reverse Geocoding. In this post I 'm gonna show you how this could be achieved with famous Google Map API. This entire post is based on one API request. Look at below code.
http://maps.googleapis.com/maps/api/geocode/json?latlng=-37.814251,144.963169&sensor=false
Enter above line in the browser bar to see a whole bunch of location details for the geographical point (-37.814251,144.963169) in lat lng format.
Pretty Simple. Here I'm using Google Map JavaScript API V3. All you need to do is to parse the json response to extract the information whatever you need.
jQuery.ajax({
url: 'http://maps.googleapis.com/maps/api/geocode/json?latlng=-37.814251,144.963169&sensor=false',
type: 'POST',
dataType: 'json',
success: function(data) {
if(data.status == 'OK')
{
alert(data.results[1].formatted_address);
alert(data.results[1].address_components[0].long_name);
alert(data.results[1].address_components[1].long_name);
}
},
error: function(xhr, textStatus, errorThrown) {
alert("Unable to resolve your location");
}
});
Google Map Street View
Demo
<!DOCTYPE html>
<html>
<head>
<meta charset="<a>utf-8</a>">
<title>Street View service</title>
<link href="<a href="view-source:https://google-developers.appspot.com/maps/documentation/javascript/examples/default.css">/maps/documentation/javascript/examples/default.css</a>" rel="<a>stylesheet</a>">
<script src="<a href="view-source:https://maps.googleapis.com/maps/api/js?v=3.exp&sensor=false">https://maps.googleapis.com/maps/api/js?v=3.exp&sensor=false</a>"></script>
<script>
function initialize() {
var fenway = new google.maps.LatLng(42.345573,-71.098326);
var mapOptions = {
center: fenway,
zoom: 14,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(
document.getElementById('map-canvas'), mapOptions);
var panoramaOptions = {
position: fenway,
pov: {
heading: 34,
pitch: 10
}
};
var panorama = new google.maps.StreetViewPanorama(document.getElementById('pano'),panoramaOptions);
map.setStreetView(panorama);
}
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="<a>map-canvas</a>" style="<a>width: 400px; height: 300px</a>"></div>
<div id="<a>pano</a>" style="<a>position:absolute; left:410px; top: 8px; width: 400px; height: 300px;</a>"></div>
</body>
</html>
Building ASP.NET MVC3 Applications
![]() |
ASP.NET MVC3 |
Welcome Back, today we gonna build a simple MVC application using ASP.net MVC3 template. The tutorial does not intend to teach you ASP.net MVC3 framework. There are so many resources on the internet that might help you getting started with this technology. So we focus on building a simple application using models, views, controllers, filters and other ASP.net specific things. Remember MVC is a design pattern and it can also be implemented in other programing languages like PHP.
Final result

Download Project
1. Creating New Project


2. Folder Structure

3. Changing the default controller.
You can change the default controller in Global.asax file by specifying the controller name and action method.

4. Adding Dojo files
We are using Dojo data grid for displaying data records. Therefore please download Dojo latest version and include them in Scripts folder.
5. Creating Book Modal
Right click on Models -> Add -> Class
Book.cs
[sourcecode language="csharp"]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace BooksApp.Models
{
public class Book
{
public int Id { get; set; }
[Required]
public string Name { get; set; }
[Required]
public string Author { get; set; }
[Required]
public float Price { get; set; }
}
}
[/sourcecode]
6. Creating BookFacade classFor a real application database operations can be placed in this class. For the sake of simplicity we omit mapping entity to database.
Right click on Models -> Add -> Class
BookFacade.cs
[sourcecode language="csharp"]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using BooksApp.Models;
namespace BooksApp.Facade
{
public class BookFacade
{
public static List<Book> bookList { get; set; }
public static List<Book> GetBookList()
{
if (bookList == null)
{
bookList = new List<Book>();
for (int i = 0; i < 10; i++)
{
bookList.Add(new Book {Id = i, Name = "Book " + i, Author = "Author " + i, Price = i * 20 });
}
}
return bookList;
}
public static Book FindBook(int id)
{
var bookList = GetBookList();
return bookList.Find(x => x.Id == id);
}
public static void Add(Book book)
{
var bookList = GetBookList();
book.Id = bookList.Max(x => x.Id) + 1;
bookList.Add(book);
}
public static void Delete(int id)
{
var bookList = GetBookList();
Book book = bookList.Find(x => x.Id == id);
bookList.Remove(book);
}
}
}
[/sourcecode]
7. Creating BookController
Right click on Controllers-> Add -> Controller
BookController
[sourcecode language="csharp"]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using BooksApp.Models;
using BooksApp.Facade;
using BooksApp.Filters;
namespace BooksApp.Controllers
{
public class BookController : Controller
{
//
// GET: /Book/
public ActionResult Index()
{
return View();
}
public ActionResult Create()
{
return View();
}
[AcceptVerbs(HttpVerbs.Post)]
public ActionResult Create(Book book)
{
if (book.Name.Length < 5)
{
ModelState.AddModelError("Book name", "Book name should have five or more characters.");
}
if (!ModelState.IsValid)
{
return View("Create",book);
}
BookFacade.Add(book);
return RedirectToAction("Index");
}
public ActionResult GetData(int id)
{
Book book = BookFacade.FindBook(id);
return View(book);
}
[RightChecker(AllowedBookIds = "5,6,7")]
public ActionResult Delete(int id)
{
Book book = BookFacade.FindBook(id);
if (book != null)
{
BookFacade.Delete(id);
}
return RedirectToAction("Index");
}
/////////////////////////AJAX/////////////////////////////
public ActionResult GetJSON()
{
return Json(BookFacade.GetBookList(), JsonRequestBehavior.AllowGet);
}
public ActionResult Ajax()
{
return View();
}
[AcceptVerbs(HttpVerbs.Post)]
public ActionResult Create_AJAX(Book book)
{
BookFacade.Add(book);
return new EmptyResult();
}
}
}
[/sourcecode]
Adding views
8. Adding Create View

[sourcecode anguage="csharp"]
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<BooksApp.Models.Book>" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Create</title>
</head>
<body>
<script src="<%: Url.Content("~/Scripts/jquery-1.5.1.min.js") %>" type="text/javascript"></script>
<script src="<%: Url.Content("~/Scripts/jquery.validate.min.js") %>" type="text/javascript"></script>
<script src="<%: Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js") %>" type="text/javascript"></script>
<% using (Html.BeginForm("Create","Book")) { %>
<%: Html.ValidationSummary(true) %>
<fieldset>
<legend>Book</legend>
<div>
<%: Html.LabelFor(model => model.Name) %>
</div>
<div>
<%: Html.EditorFor(model => model.Name) %>
<%: Html.ValidationMessageFor(model => model.Name) %>
</div>
<div>
<%: Html.LabelFor(model => model.Author) %>
</div>
<div>
<%: Html.EditorFor(model => model.Author) %>
<%: Html.ValidationMessageFor(model => model.Author) %>
</div>
<div>
<%: Html.LabelFor(model => model.Price) %>
</div>
<div>
<%: Html.EditorFor(model => model.Price)%>
<%: Html.ValidationMessageFor(model => model.Price)%>
</div>
<p>
<input type="submit" value="Create" />
</p>
</fieldset>
<% } %>
<div>
<%: Html.ActionLink("Back to List", "Index") %>
</div>
</body>
</html>
[/sourcecode]
9. Adding GetData View

[sourcecode language="csharp"]
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<BooksApp.Models.Book>" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Book Details</title>
</head>
<body>
<fieldset>
<legend>Book</legend>
<div>Name</div>
<div>
<%: Html.DisplayFor(model => model.Name) %>
</div>
<div>Author</div>
<div>
<%: Html.DisplayFor(model => model.Author) %>
</div>
<div>Price</div>
<div>
<%: Html.DisplayFor(model => model.Price) %>
</div>
</fieldset>
<p>
<%: Html.ActionLink("Edit", "Edit", new { id=Model.Id }) %> |
<%: Html.ActionLink("Back to List", "Index") %>
</p>
</body>
</html>
[/sourcecode]
10. Adding Index View
[sourcecode language="csharp"]
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<dynamic>" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Index</title>
<style type="text/css">
@import "../../Scripts/dojo/dijit/themes/dijit.css";
@import "../../Scripts/dojo/dojox/grid/resources/Grid.css";
@import "../../Scripts/dojo/dojox/grid/resources/tundraGrid.css";
@import "../../Scripts/dojo/dijit/themes/tundra/tundra.css";
</style>
<script type="text/javascript" src="../../Scripts/dojo/dojo/dojo.js"
djconfig="isDebug:false, debugAtAllCosts:false"></script>
<script src="../../Scripts/dojo/dijit/dijit.js" type="text/javascript"></script>
<script language="javascript" type="text/javascript">
dojo.require("dojox.grid.DataGrid");
dojo.require("dojo.data.ItemFileWriteStore");
dojo.ready(function () {
DisplayAll();
});
function DisplayAll() {
var that = this;
// The parameters to pass to xhrGet, the url, how to handle it, and the callbacks.
var xhrArgs = {
url: "../Book/GetJSON",
handleAs: "json",
preventCache: true,
load: function (data, ioargs) {
that.PopulateGrid(data); //This will populate the grid
},
error: function (error, ioargs) {
alert(ioargs.xhr.status);
}
}
// Call the asynchronous xhrGet
dojo.xhrGet(xhrArgs);
}
function PopulateGrid(store) {
var jsonString = "{identifier: \"Id\", items: " + dojo.toJson(store) + "}"; //Creates the Json data that supports the grid structure. The identifier value should be unique or errors will be thrown
var dataStore = new dojo.data.ItemFileReadStore({ data: dojo.fromJson(jsonString) }); //Converts it back to an object and set it as the store
/*set up layout of the grid that will be columns*/
var gridStructure = [
{ field: 'Id', name: 'Book Id', styles: 'text-align: center;', width: 20 },
{ field: 'Name', name: 'Name', width: 20 },
{ field: 'Author', name: 'Author', width: 20 },
{ field: 'Price', name: 'Price', width: 30} //"field" matches to the JSON objects field
];
/*create a new grid:*/
var bookGrid = dijit.byId('gridS');
if (bookGrid == null) { //Only create a grid if there grid already created
var grid = new dojox.grid.DataGrid({
id: 'gridS',
store: dataStore,
structure: gridStructure,
rowSelector: '30px',
height: '300px'
},
"gridDivTag"); //The div tag that is used to place the grid
/*Call startup() to render the grid*/
grid.startup();
}
else {
bookGrid._refresh();
bookGrid.setStore(dataStore); //Setting the new datastore after entering new data
}
}
</script>
</head>
<body>
<div>
<h1>Welcome to Book Management Section</h1>
<% //HtmlAnchor %>
<div id="gridDivTag"></div>
<br /><br />
<%: Html.ActionLink("Add New", "Create") %>
<%: Html.ActionLink("Ajax Call", "Ajax") %>
</div>
</body>
</html>
[/sourcecode]
11. Adding AJAX ViewI wanna create this view just to demonstrate AJAX operations with ASP.NET MVC3
[sourcecode language="csharp"]
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<dynamic>" %>
<!DOCTYPE html>
<html>
<head id="Head1" runat="server">
<title>Ajax</title>
<style type="text/css">
@import "../../Scripts/dojo/dijit/themes/dijit.css";
@import "../../Scripts/dojo/dojox/grid/resources/Grid.css";
@import "../../Scripts/dojo/dojox/grid/resources/tundraGrid.css";
@import "../../Scripts/dojo/dijit/themes/tundra/tundra.css";
.style1
{
width: 134px;
}
</style>
<script type="text/javascript" src="../../Scripts/dojo/dojo/dojo.js" djconfig="isDebug:false, debugAtAllCosts:false"></script>
<script src="../../Scripts/dojo/dijit/dijit.js" type="text/javascript"></script>
<script language="javascript" type="text/javascript">
dojo.require("dojox.grid.DataGrid");
dojo.require("dojo.data.ItemFileWriteStore");
dojo.ready(function () {
DisplayAll();
});
function book() {
this.Name = dojo.byId("name").value;
this.Author = dojo.byId("author").value;
this.Price = dojo.byId("price").value;
}
function addBook_post() {
var bookObj = new book();
var xhrArgs = {
url: "../Book/Create_AJAX",
headers: { //Adding the request headers
"Content-Type": "application/json; charset=utf-8" // This is important to model bind to the server
},
postData: dojo.toJson(bookObj, true), //Converting the object in to Json to be sent the action method
handleAs: "json",
load: function (data) {
DisplayAll();
},
error: function (error) {
alert('error');
}
}
dojo.xhrPost(xhrArgs);
}
function DisplayAll() {
var that = this;
// The parameters to pass to xhrGet, the url, how to handle it, and the callbacks.
var xhrArgs = {
url: "../Book/GetJSON",
handleAs: "json",
preventCache: true,
load: function (data, ioargs) {
that.PopulateGrid(data); //This will populate the grid
},
error: function (error, ioargs) {
alert(ioargs.xhr.status);
}
}
// Call the asynchronous xhrGet
dojo.xhrGet(xhrArgs);
}
function PopulateGrid(store) {
var jsonString = "{identifier: \"Id\", items: " + dojo.toJson(store) + "}"; //Creates the Json data that supports the grid structure. The identifier value should be unique or errors will be thrown
var dataStore = new dojo.data.ItemFileReadStore({ data: dojo.fromJson(jsonString) }); //Converts it back to an object and set it as the store
/*set up layout of the grid that will be columns*/
var gridStructure = [
{ field: 'Id', name: 'Book Id', styles: 'text-align: center;', width: 20 },
{ field: 'Name', name: 'Name', width: 20 },
{ field: 'Author', name: 'Author', width: 20 },
{ field: 'Price', name: 'Price', width: 30} //"field" matches to the JSON objects field
];
/*create a new grid:*/
var bookGrid = dijit.byId('gridS');
if (bookGrid == null) { //Only create a grid if there grid already created
var grid = new dojox.grid.DataGrid({
id: 'gridS',
store: dataStore,
structure: gridStructure,
rowSelector: '30px',
height: '300px'
},
"gridDivTag"); //The div tag that is used to place the grid
/*Call startup() to render the grid*/
grid.startup();
}
else {
bookGrid._refresh();
bookGrid.setStore(dataStore); //Setting the new datastore after entering new data
}
}
</script>
</head>
<body >
<form id="form1" runat="server">
<div>
<h1>Welcome to Book Management Section</h1>
<div id="gridDivTag"></div>
<br /><br />
<table style="width: 400px;">
<caption>
Add New Book</caption>
<tr>
<td>
Name</td>
<td>
<input id="name" type="text" />
</td>
</tr>
<tr>
<td>
Author</td>
<td>
<input id="author" type="text" />
</td>
</tr>
<tr>
<td>
Price</td>
<td>
<input id="price" type="text" />
</td>
</tr>
<tr>
<td>
</td>
<td>
<input id="add_post" type="submit" value="Add Via Post" onclick="addBook_post()" />
</td>
</tr>
</table>
<br /><br />
<%: Html.ActionLink("Add New", "Create") %> <%: Html.ActionLink("Ajax Call", "Ajax") %>
</div>
</form>
</body>
</html>
[/sourcecode]
Sample testing links
http://localhost:3616/
http://localhost:3616/Book/Create
http://localhost:3616/Book/Ajax
http://localhost:3616/Book/GetData/1
http://localhost:3616/Book/GetJSON
Obviously port number may change according to your environment.
How to use PHP session upload progress bar
** Credits goes to original author of this code
test.php
[sourcecode language="php"]
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST" && !empty($_FILES["userfile"])) {
move_uploaded_file($_FILES["userfile"]["tmp_name"], "tmp/" . $_FILES["userfile"]["name"]);
}
?>
<html>
<head>
<title>File Upload Progress Bar</title>
<style type="text/css">
#bar_blank {
border: solid 1px #000;
height: 20px;
width: 300px;
}
#bar_color {
background-color: #006666;
height: 20px;
width: 0px;
}
#bar_blank, #hidden_iframe {
display: none;
}
</style>
</head>
<body>
<div id="bar_blank">
<div id="bar_color"></div>
</div>
<div id="status"></div>
<form action="<?php echo $_SERVER["PHP_SELF"]; ?>" method="POST"
id="myForm" enctype="multipart/form-data" target="hidden_iframe">
<input type="hidden" value="myForm"
name="<?php echo ini_get("session.upload_progress.name"); ?>">
<input type="file" name="userfile"><br>
<input type="submit" value="Start Upload">
</form>
<script type="text/javascript">
function toggleBarVisibility() {
var e = document.getElementById("bar_blank");
e.style.display = (e.style.display == "block") ? "none" : "block";
}
function createRequestObject() {
var http;
if (navigator.appName == "Microsoft Internet Explorer") {
http = new ActiveXObject("Microsoft.XMLHTTP");
}
else {
http = new XMLHttpRequest();
}
return http;
}
function sendRequest() {
var http = createRequestObject();
http.open("GET", "progress.php");
http.onreadystatechange = function () { handleResponse(http); };
http.send(null);
}
function handleResponse(http) {
var response;
if (http.readyState == 4) {
response = http.responseText;
document.getElementById("bar_color").style.width = response + "%";
document.getElementById("status").innerHTML = response + "%";
if (response < 100) {
setTimeout("sendRequest()", 1000);
}
else {
toggleBarVisibility();
document.getElementById("status").innerHTML = "Done.";
}
}
}
function startUpload() {
toggleBarVisibility();
setTimeout("sendRequest()", 1000);
}
(function () {
document.getElementById("myForm").onsubmit = startUpload;
})();
</script>
</body>
</html>
[/sourcecode]
progress.php
[sourcecode language="php"]
<?php
session_start();
$key = ini_get("session.upload_progress.prefix") . "myForm";
if (!empty($_SESSION[$key])) {
$current = $_SESSION[$key]["bytes_processed"];
$total = $_SESSION[$key]["content_length"];
echo $current < $total ? ceil($current / $total * 100) : 100;
}
else {
echo 100;
}
[/sourcecode]
test.php
[sourcecode language="php"]
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST" && !empty($_FILES["userfile"])) {
move_uploaded_file($_FILES["userfile"]["tmp_name"], "tmp/" . $_FILES["userfile"]["name"]);
}
?>
<html>
<head>
<title>File Upload Progress Bar</title>
<style type="text/css">
#bar_blank {
border: solid 1px #000;
height: 20px;
width: 300px;
}
#bar_color {
background-color: #006666;
height: 20px;
width: 0px;
}
#bar_blank, #hidden_iframe {
display: none;
}
</style>
</head>
<body>
<div id="bar_blank">
<div id="bar_color"></div>
</div>
<div id="status"></div>
<form action="<?php echo $_SERVER["PHP_SELF"]; ?>" method="POST"
id="myForm" enctype="multipart/form-data" target="hidden_iframe">
<input type="hidden" value="myForm"
name="<?php echo ini_get("session.upload_progress.name"); ?>">
<input type="file" name="userfile"><br>
<input type="submit" value="Start Upload">
</form>
<script type="text/javascript">
function toggleBarVisibility() {
var e = document.getElementById("bar_blank");
e.style.display = (e.style.display == "block") ? "none" : "block";
}
function createRequestObject() {
var http;
if (navigator.appName == "Microsoft Internet Explorer") {
http = new ActiveXObject("Microsoft.XMLHTTP");
}
else {
http = new XMLHttpRequest();
}
return http;
}
function sendRequest() {
var http = createRequestObject();
http.open("GET", "progress.php");
http.onreadystatechange = function () { handleResponse(http); };
http.send(null);
}
function handleResponse(http) {
var response;
if (http.readyState == 4) {
response = http.responseText;
document.getElementById("bar_color").style.width = response + "%";
document.getElementById("status").innerHTML = response + "%";
if (response < 100) {
setTimeout("sendRequest()", 1000);
}
else {
toggleBarVisibility();
document.getElementById("status").innerHTML = "Done.";
}
}
}
function startUpload() {
toggleBarVisibility();
setTimeout("sendRequest()", 1000);
}
(function () {
document.getElementById("myForm").onsubmit = startUpload;
})();
</script>
</body>
</html>
[/sourcecode]
progress.php
[sourcecode language="php"]
<?php
session_start();
$key = ini_get("session.upload_progress.prefix") . "myForm";
if (!empty($_SESSION[$key])) {
$current = $_SESSION[$key]["bytes_processed"];
$total = $_SESSION[$key]["content_length"];
echo $current < $total ? ceil($current / $total * 100) : 100;
}
else {
echo 100;
}
[/sourcecode]
Linux Client Server Socket Program
Today let's see how to create an effective socket program with client and server communication capability. This program is based on TCP protocol. First, server program should be launched. You can specify a port in server for listening client requests. When the server get a client request, server needs to create a separate process for that client. When running client program, client should know IP address and the server port.
Server.c
[sourcecode language="c"]
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <linux/in.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
#include <stdbool.h>
#include <math.h>
float stof(const char* s);
float calculator(char *calculation);
extern int errno;
int main(int argc,char **argv)
{
int clientaddrlen, listenfd, connectfd, bytes_rcvd, listen_queue_size=1;
short int port_no;
char readBuff[1000];
struct sockaddr_in servaddr, clientaddr;
pid_t childpid;
int status;
char sendBuff[1025];
if(argc!=3){
printf("Usage Format: ./server -p <PortNumber>\n");
printf("Sample Run: ./server -p 2000\n");
exit(1);
}
port_no = atoi(argv[argc-1]);
printf("Server running at port #%d\n", port_no);
// Create server socket.
if ( (listenfd = socket(AF_INET, SOCK_STREAM, 0)) < 0){
fprintf(stderr, "Cannot create server socket! errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server socket created\n");
// Bind (attach) this process to the server socket.
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = htonl(INADDR_ANY);
servaddr.sin_port = htons(port_no);
errno = bind(listenfd, (struct sockaddr *) &servaddr, sizeof(servaddr));
if(errno < 0){
printf("Server bind failure errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server socket is bound to port #%d\n", port_no);
// Turn 'listenfd' to a listening socket. Listen queue size is 1.
errno=listen(listenfd,listen_queue_size);
if(errno < 0){
printf("Server listen failure errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server listening with a queue of size %d. \n", listen_queue_size);
// Wait for connection(s) from client(s).
while (1){
clientaddrlen = sizeof(clientaddr);
connectfd = accept(listenfd, (struct sockaddr *) &clientaddr, &clientaddrlen);
if(connectfd<0){
printf("Server accept failure errno %d: %s\n",errno,strerror(errno));
exit(1);
}
printf("A connection received from a client. Creating a child to serve the client.\n");
if((childpid = fork()) == 0) { /* child process */
close(listenfd); /* close listening socket */
printf("Child process serving the client.\n");
if (recv(connectfd, readBuff, sizeof(readBuff), 0 ) > 0){
printf("Received message: %s\n", readBuff);
float answer = calculator(readBuff);
char msg[60];
char chAns[30]="";
sprintf(chAns,"%f",answer);
strcat(msg,chAns);
write(connectfd,msg, strlen(readBuff));
}
//close(connectfd); /* parent closes connected socket */
exit(1);
}
else if (childpid <0){ /* failed to fork */
printf("Failed to fork\n");
printf("Fork error errno %d: %s\n",errno,strerror(errno));
}
else if(childpid != 0){ /* parent process */
close(connectfd); /* parent closes connected socket */
childpid = wait(&status);
}
}
return 0;
}
float calculator(char calculation[256])
{
char *token;
char *val1;
char *val2;
float v1;
float v2;
float ans=-9999.0f;
bool validCal = true;
printf("****\n");
char tempCalculation[256];
strcpy(tempCalculation,calculation);
printf("****\n");
token = strtok(tempCalculation,"+-*//^");
int c = 0;
while(token != NULL)
{
c++;
printf("%d\n",c);
if(c == 1)
val1 = token;
if(c == 2)
val2 = token;
printf("%s\n",token);
token = strtok(NULL,"+-*//^");
}
if(c == 2)
{
int i1;
const int lenVal1 = strlen(val1);
for(i1=0; i1<lenVal1 ; i1++)
{
if(!isdigit(val1[i1]))
break;
}
if(i1 != lenVal1)
validCal = false;
int i2;
const int lenVal2 = strlen(val2)-1;
for(i2=0; i2<lenVal2 ; i2++)
{
if(!isdigit(val2[i2]))
break;
}
if(i2 != lenVal2)
validCal = false;
printf("operand1:%soperand2:%s\nlen1:%dlen2%d\n",val1,val2,lenVal1,lenVal2);
if(validCal)
{
char operator = calculation[lenVal1];
printf("Operator%c\n",operator);
printf("Calculation:%s\n",calculation);
v1 = stof(val1);
v2 = stof(val2);
printf("v1=%f\n",v1);
printf("v2=%f\n",v2);
float fv1 = stof(val1);
printf("fv1=%f\n",fv1);
//float f1 = 56.4f;
//float f2 = 2.4f;
if(operator == '+')
{
printf("Addition\n");
ans = v1 + v2;
}
else if(operator == '-')
{
printf("Substraction\n");
ans = v1 - v2;
}
else if(operator == '*')
{
printf("Multiplication\n");
ans = v1 * v2;
}
else if(operator == '/')
{
printf("Devision\n");
ans = v1 / v2;
}
else if(operator == '^')
{
printf("Power\n");
}
else
{
}
printf("Answer:%f\n",ans);
}
else
{
printf("The values are not numeric!\n");
}
return ans;
}
else
{
printf("Two operands are required for calculation\n");
validCal = false;
return ans;
}
};
float stof(const char* s){
float rez = 0, fact = 1;
if (*s == '-'){
s++;
fact = -1;
};
int point_seen;
for (point_seen = 0; *s; s++){
if (*s == '.'){
point_seen = 1;
continue;
};
int d = *s - '0';
if (d >= 0 && d <= 9){
if (point_seen) fact /= 10.0f;
rez = rez * 10.0f + (float)d;
};
};
return rez * fact;
};
[/sourcecode]
Client.c
[sourcecode language="c"]
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <linux/in.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
int main(int argc,char **argv)
{
int clientfd;
short int port_no;
char msg[1000];
char buff[1000];
struct sockaddr_in servaddr;
char *server_ip_address;
if(argc!=5){
printf("Usage Format: ./client -a <IPAddress> -p <PortNumber>\n");
printf("Sample Run: ./client -a 127.0.0.1 -p 2000\n");
exit(1);
}
server_ip_address=(char *)argv[2];
port_no = atoi(argv[4]);
printf("Client will connect to the server at IP %s, port #%d\n", server_ip_address, port_no);
// Create client socket.
if((clientfd = socket(AF_INET, SOCK_STREAM, 0))<0){
printf("Socket could not be created\n");
printf("errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Client socket created\n");
errno=0;
// Connect to the server client
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(port_no);
if((inet_pton(AF_INET, server_ip_address, &servaddr.sin_addr))<=0){
printf("inet_pton error for %s\n",server_ip_address);
printf("errno %d: %s\n",errno,strerror(errno));
exit(1);
}
errno=0;
if((connect(clientfd, (struct sockaddr *) &servaddr, sizeof(servaddr)))<0){
printf("Connect error\n");
printf("errno %d: %s\n",errno,strerror(errno));
exit(1);
}
printf("Client socket connected\n");
// Read one line of message from the input and send it to the server.
while(1)
{
printf("\nEnter the message to be sent to the server: ");
scanf("%s", msg);
send(clientfd, msg, strlen(msg)+1, 0);
//close(clientfd);
if (recv(clientfd, buff, sizeof(buff), 0 ) > 0){
printf("Server Response: %s\n", buff);
}
}
return 0;
}
[/sourcecode]
Run server :
gcc -oa server.c
./a -p 2000
Run client:
gcc -ob client.c
./b -a 127.0.0.1 -p 2000
Server.c
[sourcecode language="c"]
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <linux/in.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
#include <stdbool.h>
#include <math.h>
float stof(const char* s);
float calculator(char *calculation);
extern int errno;
int main(int argc,char **argv)
{
int clientaddrlen, listenfd, connectfd, bytes_rcvd, listen_queue_size=1;
short int port_no;
char readBuff[1000];
struct sockaddr_in servaddr, clientaddr;
pid_t childpid;
int status;
char sendBuff[1025];
if(argc!=3){
printf("Usage Format: ./server -p <PortNumber>\n");
printf("Sample Run: ./server -p 2000\n");
exit(1);
}
port_no = atoi(argv[argc-1]);
printf("Server running at port #%d\n", port_no);
// Create server socket.
if ( (listenfd = socket(AF_INET, SOCK_STREAM, 0)) < 0){
fprintf(stderr, "Cannot create server socket! errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server socket created\n");
// Bind (attach) this process to the server socket.
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = htonl(INADDR_ANY);
servaddr.sin_port = htons(port_no);
errno = bind(listenfd, (struct sockaddr *) &servaddr, sizeof(servaddr));
if(errno < 0){
printf("Server bind failure errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server socket is bound to port #%d\n", port_no);
// Turn 'listenfd' to a listening socket. Listen queue size is 1.
errno=listen(listenfd,listen_queue_size);
if(errno < 0){
printf("Server listen failure errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Server listening with a queue of size %d. \n", listen_queue_size);
// Wait for connection(s) from client(s).
while (1){
clientaddrlen = sizeof(clientaddr);
connectfd = accept(listenfd, (struct sockaddr *) &clientaddr, &clientaddrlen);
if(connectfd<0){
printf("Server accept failure errno %d: %s\n",errno,strerror(errno));
exit(1);
}
printf("A connection received from a client. Creating a child to serve the client.\n");
if((childpid = fork()) == 0) { /* child process */
close(listenfd); /* close listening socket */
printf("Child process serving the client.\n");
if (recv(connectfd, readBuff, sizeof(readBuff), 0 ) > 0){
printf("Received message: %s\n", readBuff);
float answer = calculator(readBuff);
char msg[60];
char chAns[30]="";
sprintf(chAns,"%f",answer);
strcat(msg,chAns);
write(connectfd,msg, strlen(readBuff));
}
//close(connectfd); /* parent closes connected socket */
exit(1);
}
else if (childpid <0){ /* failed to fork */
printf("Failed to fork\n");
printf("Fork error errno %d: %s\n",errno,strerror(errno));
}
else if(childpid != 0){ /* parent process */
close(connectfd); /* parent closes connected socket */
childpid = wait(&status);
}
}
return 0;
}
float calculator(char calculation[256])
{
char *token;
char *val1;
char *val2;
float v1;
float v2;
float ans=-9999.0f;
bool validCal = true;
printf("****\n");
char tempCalculation[256];
strcpy(tempCalculation,calculation);
printf("****\n");
token = strtok(tempCalculation,"+-*//^");
int c = 0;
while(token != NULL)
{
c++;
printf("%d\n",c);
if(c == 1)
val1 = token;
if(c == 2)
val2 = token;
printf("%s\n",token);
token = strtok(NULL,"+-*//^");
}
if(c == 2)
{
int i1;
const int lenVal1 = strlen(val1);
for(i1=0; i1<lenVal1 ; i1++)
{
if(!isdigit(val1[i1]))
break;
}
if(i1 != lenVal1)
validCal = false;
int i2;
const int lenVal2 = strlen(val2)-1;
for(i2=0; i2<lenVal2 ; i2++)
{
if(!isdigit(val2[i2]))
break;
}
if(i2 != lenVal2)
validCal = false;
printf("operand1:%soperand2:%s\nlen1:%dlen2%d\n",val1,val2,lenVal1,lenVal2);
if(validCal)
{
char operator = calculation[lenVal1];
printf("Operator%c\n",operator);
printf("Calculation:%s\n",calculation);
v1 = stof(val1);
v2 = stof(val2);
printf("v1=%f\n",v1);
printf("v2=%f\n",v2);
float fv1 = stof(val1);
printf("fv1=%f\n",fv1);
//float f1 = 56.4f;
//float f2 = 2.4f;
if(operator == '+')
{
printf("Addition\n");
ans = v1 + v2;
}
else if(operator == '-')
{
printf("Substraction\n");
ans = v1 - v2;
}
else if(operator == '*')
{
printf("Multiplication\n");
ans = v1 * v2;
}
else if(operator == '/')
{
printf("Devision\n");
ans = v1 / v2;
}
else if(operator == '^')
{
printf("Power\n");
}
else
{
}
printf("Answer:%f\n",ans);
}
else
{
printf("The values are not numeric!\n");
}
return ans;
}
else
{
printf("Two operands are required for calculation\n");
validCal = false;
return ans;
}
};
float stof(const char* s){
float rez = 0, fact = 1;
if (*s == '-'){
s++;
fact = -1;
};
int point_seen;
for (point_seen = 0; *s; s++){
if (*s == '.'){
point_seen = 1;
continue;
};
int d = *s - '0';
if (d >= 0 && d <= 9){
if (point_seen) fact /= 10.0f;
rez = rez * 10.0f + (float)d;
};
};
return rez * fact;
};
[/sourcecode]
Client.c
[sourcecode language="c"]
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <linux/in.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
int main(int argc,char **argv)
{
int clientfd;
short int port_no;
char msg[1000];
char buff[1000];
struct sockaddr_in servaddr;
char *server_ip_address;
if(argc!=5){
printf("Usage Format: ./client -a <IPAddress> -p <PortNumber>\n");
printf("Sample Run: ./client -a 127.0.0.1 -p 2000\n");
exit(1);
}
server_ip_address=(char *)argv[2];
port_no = atoi(argv[4]);
printf("Client will connect to the server at IP %s, port #%d\n", server_ip_address, port_no);
// Create client socket.
if((clientfd = socket(AF_INET, SOCK_STREAM, 0))<0){
printf("Socket could not be created\n");
printf("errno %i: %s\n",errno,strerror(errno));
exit(1);
}
printf("Client socket created\n");
errno=0;
// Connect to the server client
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(port_no);
if((inet_pton(AF_INET, server_ip_address, &servaddr.sin_addr))<=0){
printf("inet_pton error for %s\n",server_ip_address);
printf("errno %d: %s\n",errno,strerror(errno));
exit(1);
}
errno=0;
if((connect(clientfd, (struct sockaddr *) &servaddr, sizeof(servaddr)))<0){
printf("Connect error\n");
printf("errno %d: %s\n",errno,strerror(errno));
exit(1);
}
printf("Client socket connected\n");
// Read one line of message from the input and send it to the server.
while(1)
{
printf("\nEnter the message to be sent to the server: ");
scanf("%s", msg);
send(clientfd, msg, strlen(msg)+1, 0);
//close(clientfd);
if (recv(clientfd, buff, sizeof(buff), 0 ) > 0){
printf("Server Response: %s\n", buff);
}
}
return 0;
}
[/sourcecode]
Run server :
gcc -oa server.c
./a -p 2000
Run client:
gcc -ob client.c
./b -a 127.0.0.1 -p 2000
OpenLayers - How to add markers and popups
Demo
[sourcecode language="javascript"]
<script src="http://openlayers.org/api/OpenLayers.js"></script>
<script type="text/javascript">
function init(){
map = new OpenLayers.Map('map');
base_layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(base_layer);
map.setCenter(new OpenLayers.LonLat(79.8333,6.9167),7);
var markers = new OpenLayers.Layer.Markers( "Markers" );
map.addLayer(markers);
var size = new OpenLayers.Size(21,25);
var offset = new OpenLayers.Pixel(-(size.w/2), -size.h);
var icon = new OpenLayers.Icon('http://www.openlayers.org/dev/img/marker.png', size, offset);
markers.addMarker(new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167),icon));
markers.addMarker(new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167),icon.clone()));
marker = new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167));
markers.addMarker(marker);
marker.events.register("click", marker, function(e){
popup = new OpenLayers.Popup.FramedCloud("chicken",
new OpenLayers.LonLat(79.8333,6.9167),
new OpenLayers.Size(200, 200),
"I was here <br><img src='uploads/me.png' width='90' height='90'>",
null, true);
map.addPopup(popup);
});
}
</script>
</head>
<body onLoad="init()">
<div id="map" style="width:500px;height:300px;margin-left: auto;margin-right:auto;"></div>
[/sourcecode]
[sourcecode language="javascript"]
<script src="http://openlayers.org/api/OpenLayers.js"></script>
<script type="text/javascript">
function init(){
map = new OpenLayers.Map('map');
base_layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(base_layer);
map.setCenter(new OpenLayers.LonLat(79.8333,6.9167),7);
var markers = new OpenLayers.Layer.Markers( "Markers" );
map.addLayer(markers);
var size = new OpenLayers.Size(21,25);
var offset = new OpenLayers.Pixel(-(size.w/2), -size.h);
var icon = new OpenLayers.Icon('http://www.openlayers.org/dev/img/marker.png', size, offset);
markers.addMarker(new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167),icon));
markers.addMarker(new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167),icon.clone()));
marker = new OpenLayers.Marker(new OpenLayers.LonLat(79.8333,6.9167));
markers.addMarker(marker);
marker.events.register("click", marker, function(e){
popup = new OpenLayers.Popup.FramedCloud("chicken",
new OpenLayers.LonLat(79.8333,6.9167),
new OpenLayers.Size(200, 200),
"I was here <br><img src='uploads/me.png' width='90' height='90'>",
null, true);
map.addPopup(popup);
});
}
</script>
</head>
<body onLoad="init()">
<div id="map" style="width:500px;height:300px;margin-left: auto;margin-right:auto;"></div>
[/sourcecode]
Getting the coordinates of a click in OpenLayers Map
Demo
[sourcecode language="javascript"]
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Getting the coordinates of a click in OpenLayers Map</title>
<script src="http://openlayers.org/api/OpenLayers.js"></script>
<script type="text/javascript">
function init(){
map = new OpenLayers.Map('map');
base_layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(base_layer);
map.setCenter(new OpenLayers.LonLat(79.8333,6.9167),7);
map.events.register('click', map, handleMapClick);
}
function handleMapClick(evt)
{
var lonlat = map.getLonLatFromViewPortPx(evt.xy);
alert("latitude : " + lonlat.lat + ", longitude : " + latitude);
}
</script>
</head>
<body onLoad="init()">
</body>
</html>
[/sourcecode]
[sourcecode language="javascript"]
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Getting the coordinates of a click in OpenLayers Map</title>
<script src="http://openlayers.org/api/OpenLayers.js"></script>
<script type="text/javascript">
function init(){
map = new OpenLayers.Map('map');
base_layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(base_layer);
map.setCenter(new OpenLayers.LonLat(79.8333,6.9167),7);
map.events.register('click', map, handleMapClick);
}
function handleMapClick(evt)
{
var lonlat = map.getLonLatFromViewPortPx(evt.xy);
alert("latitude : " + lonlat.lat + ", longitude : " + latitude);
}
</script>
</head>
<body onLoad="init()">
</body>
</html>
[/sourcecode]
Subscribe to:
Posts (Atom)
1 comments: