Edge Detection with OpenCV
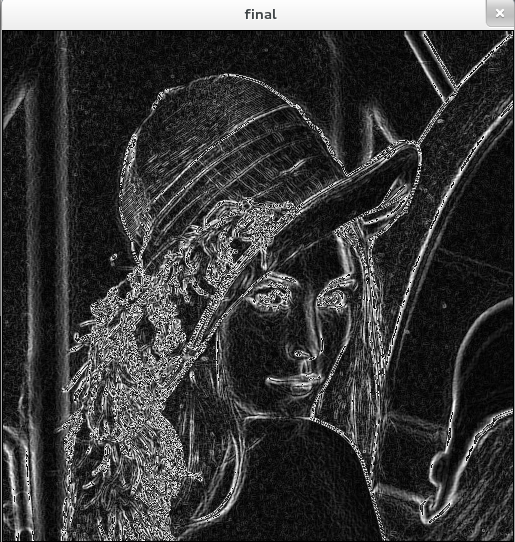
Edge detection is considered as the most common approach for detecting meaningful discontinuities in the grey- level.
1. Sobel Operator
void cvSobel( const CvArr* src, CvArr* dst, int xorder, int yorder,
int aperture_size=3 );
Parameters:
src – Source image
dst – Destination image
xorder – First Order derivative in x direction
yorder – First Order derivative in y direction
apertureSize – Size of the extended Sobel kernel, must be 1, 3, 5 or 7
The function is called with (xorder=1, yorder=0, aperture_size=3)
|-1 0 1|
|-2 0 2|
|-1 0 1|
and (xorder=0, yorder=1, aperture_size=3)
|-1 -2 -1|
| 0 0 0|
| 1 2 1|
[sourcecode language="c"]
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("building.jpg");
IplImage *gray;
IplImage *sobelX;
IplImage *sobelY;
IplImage *tempX;
IplImage *tempY;
gray =cvCreateImage(cvSize(img->width,img->height),8,1);
cvCvtColor(img,gray,CV_RGB2GRAY);
if(!img)
{
printf("Error :coudn't open the image file.\n");
return 1;
}
sobelX =cvCreateImage(cvSize(img->width,img->height),8,1);
sobelY =cvCreateImage(cvSize(img->width,img->height),8,1);
// Create Temp Images
tempX =cvCreateImage(cvSize(img->width,img->height),IPL_DEPTH_16S,1);
tempY =cvCreateImage(cvSize(img->width,img->height),IPL_DEPTH_16S,1);
cvSobel(gray,tempX,1,0,3);
cvSobel(gray,tempY,0,1,3);
// Convert to the Absolute Value
cvConvertScaleAbs(tempX,sobelX,1,0);
cvConvertScaleAbs(tempY,sobelY,1,0);
cvNamedWindow("Image:",1);
cvShowImage("Image:", img);
cvNamedWindow("Image:",1);
cvShowImage("Gray:", gray);
cvNamedWindow("sobelx:",1);
cvNamedWindow("sobely:",1);
cvShowImage("sobelx:", sobelX);
cvShowImage("sobely:", sobelY);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Gray:");
cvDestroyWindow("sobelx:");
cvDestroyWindow("sobely:");
cvReleaseImage(&img);
cvReleaseImage(&gray);
cvReleaseImage(&sobelX);
cvReleaseImage(&sobelY);
return 0;
}
[/sourcecode]
1. LaplacianOperator
The function calculates the Laplacian of the source image by filtering the image with the
following 3X3 aperture:
|-1 -1 -1|
| -1 8 -1|
|-1 -1 -1|
Use the following function to apply the Laplacian operator:
void cvLaplace(const CvArr* src, CvArr* dst, int apertureSize=3)
Parameters:
src – Source image
dst – Destination image
apertureSize – Size of the extended Sobel kernel
[sourcecode language="c"]
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("building.jpg");
IplImage *gray;
IplImage *laplace;
IplImage *temp;
gray =cvCreateImage(cvSize(img->width,img->height),8,1);
cvCvtColor(img,gray,CV_RGB2GRAY);
temp =cvCreateImage(cvSize(img->width,img->height),IPL_DEPTH_16S,1);
laplace =cvCreateImage(cvSize(img->width,img->height),8,1);
IplImage *dst1=cvCreateImage(cvSize(img->width,img->height),8,1);
cvLaplace(gray,temp,3);
cvConvertScaleAbs(temp,laplace,1,0);
cvSmooth( laplace, dst1,CV_MEDIAN,3,3);
cvNamedWindow("Image:",1);
cvShowImage("Image:", img);
//cvNamedWindow("temp:",1);
cvNamedWindow("Laplace:",1);
cvNamedWindow("remove:",1);
cvShowImage("Laplace:", laplace);
cvShowImage("remove:", dst1);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Laplace:");
cvDestroyWindow("remove:");
//cvReleaseImage(&img);
cvReleaseImage(&laplace);
cvReleaseImage(&dst1);
return 0;
}
[/sourcecode]
Thanks for posting :)
ReplyDelete