Image Morphology using OpenCV
1. Erosion
[caption id="attachment_796" align="alignnone" width="408"]
Erosion[/caption]
generally decreases the sizes of objects and removes small anomalies by subtracting objects with a radius smaller than the structuring element.
The function describes:
src
-Source image
dst
-Destination image
element
-Structuring element used for erosion. If it is NULL, a 3×3 rectangular
structuring element is used
iterations
-Number of times erosion is applied
[sourcecode language="c"]
#include "stdafx.h"
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("Image2.png");
IplImage *dest =cvCreateImage(cvSize(img->width,img->height),8,3);
IplImage *temp =cvCreateImage(cvSize(img->width,img->height),8,3);
cvErode(img,dest,NULL,2);
cvNamedWindow("Image:");
cvNamedWindow("Destination:");
cvShowImage("Image:", img);
cvShowImage("Destination:", dest);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Destination:");
cvReleaseImage(&img);
cvReleaseImage(&dest);
return 0;
}
[/sourcecode]
2. Dilation
[caption id="attachment_798" align="alignnone" width="404"]
Dilation[/caption]
generally increases the sizes of objects, filling in holes and broken areas, and connecting areas that are separated by spaces smaller than the size of the structuring element.
[caption id="attachment_796" align="alignnone" width="408"]
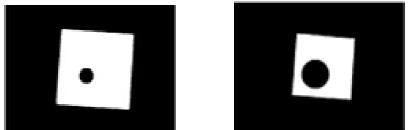
generally decreases the sizes of objects and removes small anomalies by subtracting objects with a radius smaller than the structuring element.
void cvErode( const CvArr* src, CvArr* dst,
IplConvKernel*element=NULL, int iterations=1 );
The function describes:
src
-Source image
dst
-Destination image
element
-Structuring element used for erosion. If it is NULL, a 3×3 rectangular
structuring element is used
iterations
-Number of times erosion is applied
[sourcecode language="c"]
#include "stdafx.h"
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("Image2.png");
IplImage *dest =cvCreateImage(cvSize(img->width,img->height),8,3);
IplImage *temp =cvCreateImage(cvSize(img->width,img->height),8,3);
cvErode(img,dest,NULL,2);
cvNamedWindow("Image:");
cvNamedWindow("Destination:");
cvShowImage("Image:", img);
cvShowImage("Destination:", dest);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Destination:");
cvReleaseImage(&img);
cvReleaseImage(&dest);
return 0;
}
[/sourcecode]
2. Dilation
[caption id="attachment_798" align="alignnone" width="404"]
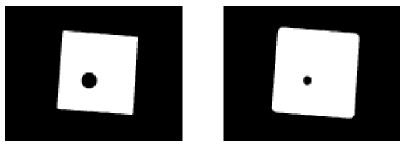
generally increases the sizes of objects, filling in holes and broken areas, and connecting areas that are separated by spaces smaller than the size of the structuring element.
void cvDilate( const CvArr* src, CvArr* dst, IplConvKernel*
element=NULL, int iterations=1 );
[sourcecode language="c"]
#include "stdafx.h"
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("Image2.png");
IplImage *dest =cvCreateImage(cvSize(img->width,img->height),8,3);
IplImage *temp =cvCreateImage(cvSize(img->width,img->height),8,3);
cvDilate(img,dest,NULL,2);
cvNamedWindow("Image:");
cvNamedWindow("Destination:");
cvShowImage("Image:", img);
cvShowImage("Destination:", dest);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Destination:");
cvReleaseImage(&img);
cvReleaseImage(&dest);
return 0;
}
[/sourcecode]
3. MorphologyEx
This function performs advanced morphological transformations, which enables composite operations such as Opening, Closing, Morphological Gradient, Top Hat and Black Hat. The one function that lets you do all this is:
void cvMorphologyEx( const CvArr* src, CvArr* dst, CvArr* temp,
IplConvKernel* element, int operation, int iterations=1 );
The function describes:
src
-Source image
dst
-Destination image
temp
-Temporary image, required in some cases
element
-Structuring element
operation
-Type of morphological operation, one of:
CV_MOP_OPEN - opening
CV_MOP_CLOSE - closing
CV_MOP_GRADIENT - morphological gradient
CV_MOP_TOPHAT - "top hat"
CV_MOP_BLACKHAT - "black hat"
iterations
-Number of times erosion and dilation are applied
[sourcecode language="c"]
#include "stdafx.h"
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
int _tmain(int argc, _TCHAR* argv[])
{
IplImage *img = cvLoadImage("Image2.png");
IplImage *dest =cvCreateImage(cvSize(img->width,img->height),8,3);
IplImage *temp =cvCreateImage(cvSize(img->width,img->height),8,3);
cvMorphologyEx(img,dest,temp,structure,MOP_CLOSE,1);
cvNamedWindow("Image:");
cvNamedWindow("Destination:");
cvShowImage("Image:", img);
cvShowImage("Destination:", dest);
cvWaitKey(0);
cvDestroyWindow("Image:");
cvDestroyWindow("Destination:");
cvReleaseImage(&img);
cvReleaseImage(&dest);
return 0;
}
[/sourcecode]
0 comments: